数据库使用
在联犀中数据库的相关操作都放到了 share/stores
中
其中我们需要重点关注: share/stores/conn.go
# 初始化
- 在配置结构体中添加数据库定义
internal/config/config.go
Database conf.Database
示例:
package config
import (
"gitee.com/unitedrhino/share/conf"
"github.com/zeromicro/go-zero/core/stores/cache"
"github.com/zeromicro/go-zero/rest"
)
type Config struct {
rest.RestConf
CacheRedis cache.ClusterConf
Event conf.EventConf //和things内部交互的设置
DmRpc conf.RpcClientConf `json:",optional"`
SysRpc conf.RpcClientConf `json:",optional"`
Database conf.Database
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
- 配置文件中添加配置,注意,修改为正确的账号密码及ip端口
Database:
DBType: mysql
DSN: root:password@tcp(127.0.0.1:3306)/iThings?charset=utf8mb4&collation=utf8mb4_bin&parseTime=true&loc=Asia%2FShanghai
1
2
3
2
3
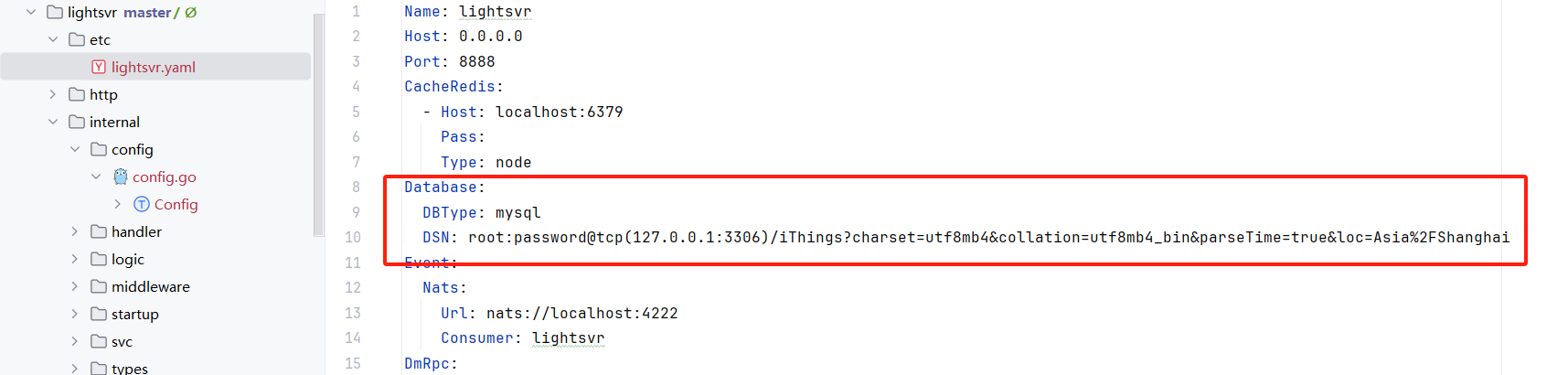
- 数据库连接初始化
在联犀中数据库连接由 stores包进行管理,位置在share/stores/conn.go
我们在业务的svc下的 serviceContext.go
在 NewServiceContext
函数中添加
stores.InitConn(c.Database)
1
即可初始化数据库连接
- 创建orm模型
在
internal/repo/relationDB/model.go
中编辑好gorm的模型,如果没有这个文件创建对应的文件,如果是数据库表可以通过转换工具 (opens new window)转换成go的结构体
创建的模型示例如下:
package relationDB
import (
"gitee.com/unitedrhino/share/stores"
"time"
)
type LightExample struct {
ID int64 `gorm:"column:id;type:bigint;primary_key;AUTO_INCREMENT"` // id编号
}
// 设备信息表
type LightDeviceMsgCount struct {
ID int64 `gorm:"column:id;type:bigint;primary_key;AUTO_INCREMENT"`
Num int64 `gorm:"column:num;type:bigint;default:0"` //数量
Date time.Time `gorm:"column:date;NOT NULL;uniqueIndex:date_type"` //统计的日期
stores.OnlyTime
}
func (m *LightDeviceMsgCount) TableName() string {
return "light_device_msg_count"
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
建议以服务名作为命名的前缀及表的前缀
引入操作模版(第一次需要) 将
things/service/dmsvr/internal/repo/relationDB/example.go
复制到业务相同路径下, 然后将文件关键词替换 Dm -> 服务名(如light)编辑数据库操作函数 复制
example.go
为deviceMsgCount.go
在deviceMsgCount.go
中将 Example 替换为DeviceMsgCount
在todo中完善过滤条件即可在业务中使用
使用示例:
//普通使用模式
err := relationDB.NewDeviceMsgCountRepo(l.ctx).Insert(l.ctx, &relationDB.LightDeviceMsgCount{
Num: 6,
Date: time.Now(),
})
//事务示例
err=stores.GetCommonConn(l.ctx).Transaction(func(tx *gorm.DB) error {
//初始化的时候一定要传tx,如果传的是ctx,则不会在这个事务里
po,err:=relationDB.NewDeviceMsgCountRepo(tx).FindOne(l.ctx,66)
if err!=nil{
return err
}
po.Num++
return relationDB.NewDeviceMsgCountRepo(tx).Update(l.ctx,po)
})
return err
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
- 数据初始化
在
lightsvr/internal/repo/relationDB/modelMigrate.go
创建对应的文件,文件内容如下:
package relationDB
import (
"context"
"gitee.com/unitedrhino/share/conf"
"gitee.com/unitedrhino/share/stores"
)
func Migrate(c conf.Database) error {
if c.IsInitTable == false {
return nil
}
db := stores.GetCommonConn(context.TODO())
err := db.AutoMigrate(
&LightDeviceMsgCount{},//需要初始化的表放这里会自动处理
)
if err != nil {
return err
}
return err
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
然后在lightsvr/internal/svc/serviceContext.go
的 NewServiceContext
中添加以下初始化函数
err:=relationDB.Migrate(c.Database)
logx.Must(err)
1
2
2
# 高级
分布式事务: share/stores/barrier/barrier.go 灵活查询: share/stores/cmp.go 时间类型: share/stores/time.go
上次更新: 2024/11/12, 13:18:12